쨍쨍
#1. AI Smart Home_LoginActivity 본문
[ 로그인 화면 ]
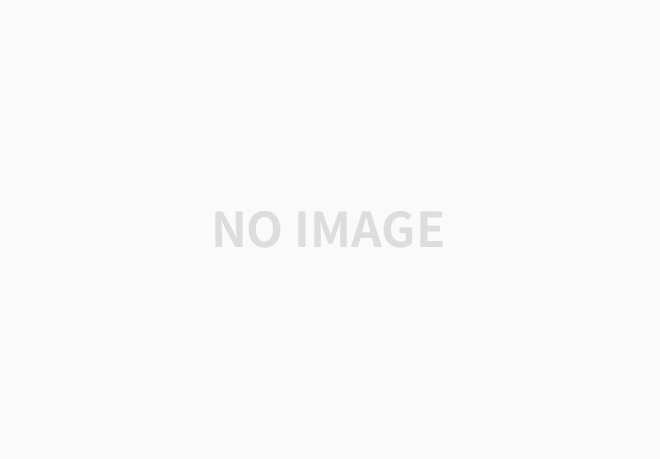
[ activitiy_login.xml ]
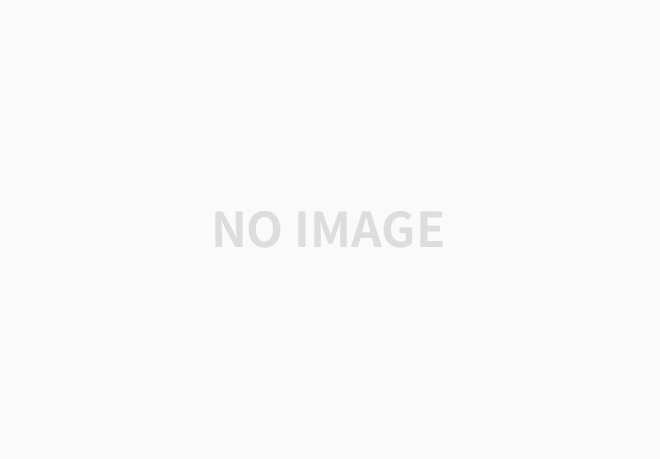
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/activity_main"
android:layout_width="match_parent"
android:layout_height="match_parent">
<!-- tools:context="com.dowellcomputer.registeration.activity_login"-->
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@color/Primary"
android:orientation="vertical">
<LinearLayout
android:layout_marginTop="30dp"
android:layout_marginLeft="30dp"
android:layout_width="350dp"
android:layout_height="600dp"
android:background="@drawable/ground"
android:orientation="vertical">
<LinearLayout
android:layout_width="match_parent"
android:background="@color/white"
android:layout_marginTop="50dp"
android:layout_height="225dp"
android:orientation="vertical">
<ImageView
android:layout_width="125dp"
android:layout_height="125dp"
android:layout_gravity="center"
android:layout_marginTop="20dp"
android:src="@drawable/ikon1"/>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:text="Ai Smart Home"
android:textColor="#FF000000"
android:textSize="25dp"
android:textStyle="bold"
android:layout_marginTop="10dp"/>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:text="로그인"
android:textColor="#FF000000"
android:textSize="18dp"
android:textStyle="bold" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<EditText
android:id="@+id/username"
android:layout_width="280dp"
android:layout_height="wrap_content"
android:hint="아이디"
android:layout_gravity="center"
android:textColor="#ffffff"
android:textColorHint="#ffffff"
android:drawableStart="@drawable/baseline_person_24"
android:drawableLeft="@drawable/baseline_person_24"
android:layout_marginTop="50dp"
android:background="@color/Primary"/>
<EditText
android:id="@+id/password"
android:inputType="textPassword"
android:layout_width="280dp"
android:layout_height="wrap_content"
android:hint="비밀번호"
android:layout_gravity="center"
android:textColor="#ffffff"
android:textColorHint="#ffffff"
android:drawableStart="@drawable/baseline_https_24"
android:drawableLeft="@drawable/baseline_https_24"
android:layout_marginTop="10dp"
android:background="@color/Primary"/>
<Button
android:id="@+id/login_button"
android:layout_width="280dp"
android:layout_height="wrap_content"
android:textSize="20dp"
android:textStyle="bold"
android:textColor="#ffffff"
android:background="@color/Button"
android:text="로그인"
android:layout_marginTop="10dp"
android:layout_gravity="center"/>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:textSize="18dp"
android:layout_marginTop="10dp"
android:text="회원가입"
android:id="@+id/registerButton"/>
</LinearLayout>
</LinearLayout>
</LinearLayout>
</androidx.constraintlayout.widget.ConstraintLayout>
[ LoginActivitiy.java ]
import android.content.Intent;
import android.os.Bundle;
import android.util.Log;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import android.widget.Toast;
import androidx.appcompat.app.AppCompatActivity;
public class LoginActivity extends AppCompatActivity {
private static final String TAG = "LoginActivity"; // TAG를 정의
private EditText usernameEditText;
private EditText passwordEditText;
private Button loginButton;
private TextView registerButton;
private SocketActivity socketActivity;
private boolean isLoggingIn = false; // 로그인 중 상태 변수
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_login);
usernameEditText = findViewById(R.id.username);
passwordEditText = findViewById(R.id.password);
loginButton = findViewById(R.id.login_button);
registerButton = findViewById(R.id.registerButton);
socketActivity = SocketActivity.getInstance();
socketActivity.connectToServer(data -> {
runOnUiThread(() -> {
loginButton.setEnabled(true); // 서버 응답 후 버튼 다시 활성화
handleServerResponse(data.trim()); // 응답 처리 메서드 호출
});
});
registerButton.setOnClickListener(view -> {
Intent registerIntent = new Intent(LoginActivity.this, RegisterActivity.class);
startActivity(registerIntent);
});
loginButton.setOnClickListener(v -> {
String username = usernameEditText.getText().toString();
String password = passwordEditText.getText().toString();
// 로그인 버튼 클릭 시 입력 데이터 유효성 검사
if (isInputValid(username, password) && !isLoggingIn) {
isLoggingIn = true; // 로그인 시도 중 상태 설정
loginButton.setEnabled(false); // 요청 중 버튼 비활성화
attemptLogin(username, password); // 로그인 시도
}
});
}
// 입력 데이터 유효성 검사 메서드
private boolean isInputValid(String username, String password) {
if (username.isEmpty()) {
Toast.makeText(this, "사용자 이름을 입력하세요.", Toast.LENGTH_SHORT).show();
return false;
}
if (password.isEmpty()) {
Toast.makeText(this, "비밀번호를 입력하세요.", Toast.LENGTH_SHORT).show();
return false;
}
return true;
}
private void attemptLogin(String username, String password) {
if (!socketActivity.isSocketConnected()) {
socketActivity.connectToServer(data -> {
if (data.equalsIgnoreCase("Connection failed")) {
runOnUiThread(() -> {
Toast.makeText(LoginActivity.this, "서버 연결 실패. 네트워크 상태를 확인해주세요.", Toast.LENGTH_SHORT).show();
loginButton.setEnabled(true);
isLoggingIn = false; // 로그인 시도 중 상태 해제
});
} else {
// 연결 성공 시 로그인 데이터 전송
socketActivity.sendLoginData(username, password);
}
});
} else {
// 연결된 경우 바로 로그인 데이터 전송
socketActivity.sendLoginData(username, password);
}
}
// 서버 응답 처리 메서드
private void handleServerResponse(String response) {
runOnUiThread(() -> {
if (isLoggingIn) {
switch (response) {
case "LOGIN_SUCCESS":
isLoggingIn = false; // 로그인 성공 후 상태 해제
Log.d(TAG, "Login successful, processing success...");
Toast.makeText(LoginActivity.this, "Login successful", Toast.LENGTH_SHORT).show();
// 로그인 성공 후 UI 갱신 및 MainActivity로 전환
Intent mainIntent = new Intent(LoginActivity.this, MainActivity.class);
startActivity(mainIntent);
finish(); // 현재 LoginActivity 종료
break;
case "Login Failed":
isLoggingIn = false; // 로그인 실패 후 상태 해제
Log.d(TAG, "Login failed.");
Toast.makeText(LoginActivity.this, "아이디 혹은 비밀번호를 확인해주세요.", Toast.LENGTH_SHORT).show();
loginButton.setEnabled(true); // 버튼 다시 활성화
break;
case "REGISTER_FAILURE: Invalid Registration Data":
Toast.makeText(LoginActivity.this, "등록된 사용자 정보가 없습니다. 회원가입을 먼저 진행해 주세요.", Toast.LENGTH_SHORT).show();
break;
case "Connection failed":
Toast.makeText(LoginActivity.this, "서버 연결 실패. 네트워크 상태를 확인해주세요.", Toast.LENGTH_SHORT).show();
break;
default:
isLoggingIn = false; // 예기치 않은 응답 시 상태 해제
Log.d(TAG, "Unexpected response: " + response);
Toast.makeText(LoginActivity.this, "Unexpected response from server: " + response, Toast.LENGTH_SHORT).show();
break;
}
}
});
}
@Override
protected void onDestroy() {
super.onDestroy();
socketActivity.disconnect();
}
}
'프로그래밍 코드 > Android Studio' 카테고리의 다른 글
#3. AI Smart Home_MainActivity (1) | 2024.03.13 |
---|---|
#2. AI Smart Home_RegisterActivity (2) | 2024.03.13 |
[Android Studio] 클릭 이벤트를 사용하는 4가지 방법 (0) | 2023.05.22 |
[Android Studio] 뷰 바인딩(View Binding) (1) | 2023.05.17 |
[Android Studio] 레이아웃 (0) | 2023.05.16 |